Property Class
This class is used to create properties that allow to control game logic-related parameter values and states. They are stored in a separate XML file with a .prop extension and can be adjusted in the editor as well. When a property parameter value is modified for a single node, an instanced property is saved into the .world, but it only contains the modified parameter rather than a whole parameters list.
To create a property, do the following:
- Manually create an XML file with .prop extension. Properties use inheritance: a child property overrides some parameters of its parent or adds new ones, while all other parameters stay the same as specified in the parent property. For example:
Source code (XML)
<?xml version="1.0" encoding="utf-8"?> <properties version="1.00"> <property editable="0" name="GameObjectsUnit"> <state name=weapon_type" type="switch" items="air,land,all_types">0</state> <state name="attack" type="toggle">1</state> <parameter name="damage" type="int" max="1000">1</parameter> <parameter name="velocity" type="float" max="100">30</parameter> <parameter name="material" type="string"/> </property> <property editable="1" name="GameObjectsNavy" parent="GameObjectUnit"> <state name="weapon_type">2</state> <parameter name="damage">120</parameter> <parameter name="material"/>military_navy</parameter> <parameter name="material_color" type="color"/>1.0 1.0 1.0 1.0</parameter> </property> </properties>
- Set the order of loading of properties. It is important when properties are stored in several files, because child properties cannot load before their parents. This can be done in via the script using engine.properties.addWorldLibrary_string():
Source code (UnigineScript)Or the properties to load and their order can be specified directly in the .world file:
engine.properties.addWorldLibrary("project/game_objects.prop"); engine.properties.addWorldLibrary("project/game_objects_unit.prop"); engine.properties.addWorldLibrary("project/game_objects_unit_weapon.prop");
Source code (XML)<world version="1.11"> <materials> ... </materials> <properties> <library>project/game_objects.prop</library> <library>project/game_objects_unit.prop</library> <library>project/game_objects_unit_weapon.prop</library> </properties> ...
Here, the created child property overrides some of the parent parameters and states and adds a new one:
Property states
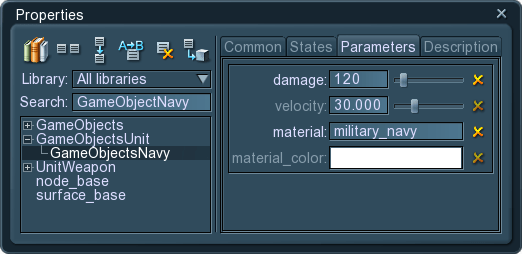
Property parameters
Property Class
Members
Property clone (string name)
Clones the property.Arguments
- string name - New property name.
Return value
Cloned property.int findParameter (string name)
Searches for a parameter by a name among all parameters of the current property.Arguments
- string name - Name of the parameter.
Return value
ID number of the parameter, if it is found; otherwise, -1.int findParameter (string name, int fast_id)
Searches for a parameter by a name and a fast identifier among all parameters of the current property.Arguments
- string name - Name of the parameter.
- int fast_id - Parameter's fast identifier (hash of a name).
Return value
ID number of the parameter, if it is found.int findState (string name)
Searches for a state by a name among all states of the current property.Arguments
- string name - Name of the parameter.
Return value
ID number of the parameter, if it is found; otherwise, -1.int findState (string name, int fast_id)
Searches for a state by a name and a fast identifier among all states of the current property.Arguments
- string name - Name of the state.
- int fast_id - State's fast identifier (hash of a name).
Return value
ID number of the state, if it is found; otherwise, -1.Property getChild (int num)
Returns a child property with a given number.Arguments
- int num - Number of the target child property.
Return value
Child property.int getCollision ()
Returns a value indicating if collision test is enabled for objects with the assigned property. The object will collide only if it has collider node flag, surface collision flag and property collision flag at the same time.Return value
Positive number if collision test is enabled; otherwise, 0.Property getCompare ()
Returns the parent property, which has been changed.Return value
Parent property.int getIntersection ()
Returns a value indicating if intersection test is enabled for objects with the assigned property. The object will collide only if it has surface intersection flag and property intersection flag at the same time.Return value
Positive number if intersection test is enabled; otherwise, 0.string getName ()
Returns the property name.Return value
Name of the property.int getNumChildren ()
Returns the number of children of the property.Return value
Number of children properties.int getNumChilds ()
Returns the number of children of the property.Warning
The function is deprecated. It is provided to keep your code working until the next release. Please, replace it with getNumChildren().
Return value
Number of children properties.int getNumParameters ()
Returns the number of property parameters.Return value
Number of property parameters.int getNumStates ()
Returns the number of property states.Return value
Number of property states.vec4 getParameterColor (int num)
Returns the current color of a given color parameter.Arguments
- int num - Number of the target parameter.
Return value
Current color.double getParameterDoubleMaxValue (int num)
Returns the maximum allowed value of a double parameter.Arguments
- int num - Number of the target parameter.
Return value
Maximum value.double getParameterDoubleMinValue (int num)
Returns the minimum allowed value of a double parameter.Arguments
- int num - Number of the target parameter.
Return value
Minimum value.double getParameterDouble (int num)
Returns the current value of a given double parameter.Arguments
- int num - Number of the target parameter.
Return value
Current value.float getParameterFloatMaxValue (int num)
Returns the maximum allowed value of a float parameter.Arguments
- int num - Number of the target parameter.
Return value
Maximum value.float getParameterFloatMinValue (int num)
Returns the minimum allowed value of a float parameter.Arguments
- int num - Number of the target parameter.
Return value
Minimum value.float getParameterFloat (int num)
Returns the current value of a given float parameter.Arguments
- int num - Number of the target parameter.
Return value
Current value.int getParameterIntMaxValue (int num)
Returns the maximum allowed value of an integer parameter.Arguments
- int num - Number of the target parameter.
Return value
Maximum value.int getParameterIntMinValue (int num)
Returns the minimum allowed value of an integer parameter.Arguments
- int num - Number of the target parameter.
Return value
Minimum value.int getParameterInt (int num)
Returns the current value of a given float parameter.Arguments
- int num - Number of the target parameter.
Return value
Current value.int getParameterMask (int num)
Returns the current value of a given mask parameter.Arguments
- int num - Number of the target parameter in range from 0 to the total number of parameters.
Return value
Current value of the parameter.string getParameterName (int num)
Returns the name of a given parameter.Arguments
- int num - Number of the target parameter.
Return value
Name of the parameter.int getParameterSliderLog10 (int num)
Returns a value indicating if a given parameter uses a logarithmic scale (with the base ten).Arguments
- int num - Number of the target parameter.
Return value
Positive number if the parameter uses a logarithmic scale; otherwise, 0.int getParameterSliderMaxExpand (int num)
Returns a value indicating if the maximum value of a given parameter can be increased.Arguments
- int num - Number of the target parameter.
Return value
Positive number if the maximum value can be changed; otherwise, 0.int getParameterSliderMinExpand (int num)
Returns a value indicating if the minimum value of a given parameter can be decreased.Arguments
- int num - Number of the target parameter.
Return value
Positive number if the minimum value can be changed; otherwise, 0.int getParameterStringFile (int num)
Returns a value indicating if a given string parameter has a file flag. For example, it is used in the Properties Editor: it allows you to specify the file for the parameter by the double-click.Arguments
- int num - Number of the target parameter.
Return value
1 if the target parameter has a file flag; otherwise 0.string getParameterString (int num)
Returns the current value of a given string parameter.Arguments
- int num - Number of the target parameter.
Return value
Current value.string getParameterSwitchItem (int num, int item)
Returns a string value for a switch item of a given parameter.Arguments
- int num - Number of the target parameter.
- int item - Number of the target item.
Return value
Value of the item or NULL (0), if an error occurred.int getParameterSwitchNumItems (int num)
Returns the number of switch items of a given parameter.Arguments
- int num - Number of the target parameter.
Return value
Number of switch items.int getParameterSwitch (int num)
Returns the current value of a given switch parameter.Arguments
- int num - Number of the target parameter.
Return value
Switch parameter value.int getParameterToggle (int num)
Returns the current value of a given toggle parameter.Arguments
- int num - Number of the target parameter.
Return value
Toggle parameter value.int getParameterType (int num)
Returns the type of a given parameter.Arguments
- int num - Number of the target parameter.
Return value
One of the PROPERTY_PARAMETER_* pre-defined variables or -1, if an error occurred.vec3 getParameterVec3 (int num)
Returns the current value of a given vec3 parameter.Arguments
- int num - Number of the target parameter.
Return value
Current value.vec4 getParameterVec4 (int num)
Returns the current value of a given vec4 parameter.Arguments
- int num - Number of the target parameter.
Return value
Current value.variable getParameter (variable variable)
Returns the value of a given parameter.Arguments
- variable variable - Parameter to be updated. It can either be:
- int - Number of the target parameter.
- string - name of the target parameter.
Return value
Parameter value.variable getParameter (variable variable, variable value)
Returns the value of a given parameter or a custom value if the specified parameter is not found.Arguments
- variable variable - Parameter to be updated. It can either be:
- int - Number of the target parameter.
- string - name of the target parameter.
- variable value - Custom value to be returned if a parameter is not found.
Return value
Parameter value or a custom value.Property getParent ()
Returns the parent property.Return value
Parent property or NULL (0), if the current property has no parent.string getStateName (int num)
Returns the name of a given state.Arguments
- int num - Number of the target state.
Return value
Name of the state.string getStateSwitchItem (int state, int item)
Returns a string value for a switch item of a given state.Arguments
- int state - Number of the target state.
- int item - Number of the target item.
Return value
Value of the item or NULL (0), if an error occurred.int getStateSwitchNumItems (int num)
Returns the number of switch items of a given state.Arguments
- int num - Number of the target state.
Return value
Number of switch items.int getStateType (int num)
Returns the type of a given state.Arguments
- int num - Number of the target state.
Return value
One of the PROPERTY_STATE_* pre-defined variables or -1, if an error occurred.int getState (variable variable)
Returns the value of the given state.Arguments
- variable variable - State to be updated. It can either be:
- int - Number of the target state.
- string - name of the target state.
Return value
State value.Property inherit (string name)
Creates a new property with a specified name and sets the current property as its parent.Arguments
- string name - New property name.
Return value
Inherited property.int isEditable ()
Returns a value indicating if the property can be edited.Return value
1 if the property is editable; otherwise, 0.int isHidden ()
Returns a value indicating if the property is hidden.Return value
1 if the property is hidden; otherwise, 0.int isParameterHidden (int num)
Returns a value indicating if a given parameter is hidden.Arguments
- int num - Number of the target parameter.
Return value
1 if the parameter is hidden; otherwise, 0.int isParent (string name)
Returns a value indicating if the property is parent.Arguments
- string name - Name of the property.
Return value
1 if the property is parent, otherwise - 0.int isStateHidden (int num)
Returns a value indicating if a given state is hidden.Arguments
- int num - Number of the target state.
Return value
1 if the state is hidden; otherwise, 0.int restoreState (Stream stream, int forced = 0)
Restores the state of a given property (all of its options, states and parameters) from a binary stream.Arguments
- Stream stream - The stream with saved property data.
- int forced - Forced restoring of property settings.
Return value
1 if the property state is restored successfully; otherwise, 0.int saveState (Stream stream, int forced = 0)
Saves the state of the given property (all of its options, states and parameters) into a binary stream.Arguments
- Stream stream - The stream to save property state data.
- int forced - Forced saving of property settings.
Return value
1 if the property state is saved successfully; otherwise, 0.void setCollision (int mode)
Updates a value indicating if collision test is enabled for objects with the assigned property. The object will collide only if it has collider node flag, surface collision flag and property collision flag at the same time.Arguments
- int mode - 1 to enable collision test, 0 to disable.
void setIntersection (int mode)
Updates a value indicating if intersection test is enabled for objects with the assigned property. The object will collide only if it has surface intersection flag and property intersection flag at the same time.Arguments
- int mode - Positive number to enable intersection test, 0 to disable.
void setParameterColor (int num, vec4 value)
Updates the color of a given color parameter.Arguments
- int num - Number of the target parameter.
- vec4 value - New color value.
void setParameterDouble (int num, double value)
Updates the value of a given double parameter.Arguments
- int num - Number of the target parameter.
- double value - New value of the parameter.
void setParameterFloat (int num, float value)
Updates the value of a given float parameter.Arguments
- int num - Number of the target parameter.
- float value - New value of the parameter.
void setParameterInt (int num, int value)
Updates the value of a given int parameter.Arguments
- int num - Number of the target parameter.
- int value - New value of the parameter.
void setParameterMask (int num, int value)
Updates the value of a given mask parameter.Arguments
- int num - Number of the target parameter in range from 0 to the total number of parameters.
- int value - New value of the mask parameter.
void setParameterString (int num, string value)
Updates the value of a given string parameter.Arguments
- int num - Number of the target parameter.
- string value - New value of the parameter.
void setParameterSwitch (int num, int value)
Updates the value of a given switch parameter.Arguments
- int num - Number of the target parameter.
- int value - New value of the parameter.
void setParameterToggle (int num, int value)
Updates the value of a given toggle parameter.Arguments
- int num - Number of the target parameter.
- int value - New value of the parameter.
void setParameterVec3 (int num, vec3 value)
Updates the value of a given vec3 parameter.Arguments
- int num - Number of the target parameter.
- vec3 value - New value of the parameter.
void setParameterVec4 (int num, vec4 value)
Updates the value of a given vec4 parameter.Arguments
- int num - Number of the target parameter.
- vec4 value - New value of the parameter.
void setParameter (variable variable, variable value)
Updates the value of a given parameter.Arguments
- variable variable - Parameter to be updated. It can either be:
- int - Number of the target parameter.
- string - name of the target parameter.
- variable value - New value of the parameter.
void setState (variable variable, int value)
Updates the value of the given state.Arguments
- variable variable - State to be updated. It can either be:
- int - Number of the target state.
- string - name of the target state.
- int value - New value of the state.
int PROPERTY_PARAMETER_AUX
Description
Parameter of the auxiliary type.int PROPERTY_PARAMETER_COLOR
Description
Parameter of this type allows specifying the material color.int PROPERTY_PARAMETER_DOUBLE
Description
Parameter of this type accepts any double value in a given range.int PROPERTY_PARAMETER_FLOAT
Description
Parameter of this type accepts any float value in a given range.int PROPERTY_PARAMETER_INT
Description
Parameter of this type accepts any integer value in a given range.int PROPERTY_PARAMETER_MASK
Description
Parameter of this type allows specifying a mask.int PROPERTY_PARAMETER_STRING
Description
Parameter of this type accepts any string value.int PROPERTY_PARAMETER_SWITCH
Description
Parameter of this type allows specifying a set of several possible values (more than two).int PROPERTY_PARAMETER_TOGGLE
Description
Parameter of this type allows only two possible values.int PROPERTY_PARAMETER_VEC3
Description
Parameter of this type accepts any vec3 value.int PROPERTY_PARAMETER_VEC4
Description
Parameter of this type accepts any vec4 value.int PROPERTY_STATE_AUX
Description
State of the auxiliary type.int PROPERTY_STATE_SWITCH
Description
State of this type allows specifying a set of several possible values (more than two).int PROPERTY_STATE_TOGGLE
Description
State of this type allows only two possible values.Last update: 03.07.2017
Помогите сделать статью лучше
Была ли эта статья полезной?
(или выберите слово/фразу и нажмите Ctrl+Enter